Class 2 |
Intermediate Java 30-IT-397 |
|
Catching Exceptions
-
If an exception is not caught, it will terminate the program.
-
Catching an exception requires a try-catch block. You must wrap the
exception-prone code in this block.
-
It starts with try {
-
It ends with } catch
-
All code that might throw an exception, such as calls to exception-throwing
methods, must go between these curly braces.
-
You can have just one catch, or multiple catches. Multiple catches
only require one try.
-
Make sure you go from most general to most specific.
-
You can even nest try-catches.
-
Over-simplified examples (these are all legal):
try {
someMethod();
} catch (Exception e) {
System.out.println(e.getMessage());
e.printStackTrace();
}
try {
someMethod1();
try {
someMethod2();
System.out.println("Print
Something Meaningless");
} catch (Exception e) {
e.printStackTrace();
}
} catch (FileNotFoundException f) {
f.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
-
When an exception is thrown, it skips all of the code between it and the
catch block. So if someMethod2 threw an exception, the System.out.println()
line would not print.
-
If you do not have a handler for an exception or one of its superclasses,
it acts as if there is no exception handler at all. It will end the
program.
-
You can re-throw an exception in a class. This throws it to the calling
method. Example:
} catch (Exception e) {
System.out.println("Exception caught.
Re-throwing.");
throw e;
}
-
If you don't have a catch block, you can pass the exception along by declaring
that exception in the signature of the calling method. This passes
up the exception indefinitely until it finds a catch block. Sometimes
this is the best approach.
Finally
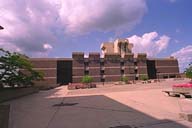
Created by: Brandan Jones
December 17, 2001