Class 2 |
Intermediate Java 30-IT-397 |
|
Behavioral Patterns
-
Resource: http://www.patterndepot.com/put/8/Behavioral.html
-
Behavioral patterns describe communication among objects.
-
The command pattern
is my favorite. It allows an object to call a method of another object,
without knowing what will really take place. This is ideal for something
that you want to re-use over multiple applications.
-
For example, say you want to have a login screen that you want to use in
many different parts of a program or web page. What will happen when
the user clicks 'Login'? In traditional programming, you might have
a complex if test that tells the program what page or screen to display
next. But the command pattern lets you do something different.
-
You can have the calling screen or page call the login screen and pass
it an object that implements an interface. Let's say this interface
has one method, called login(). When the user clicks the login button,
this method is called. What happens next depends upon what is in
the login() method of the object that was passed in by the first screen.
Thus, no if tests, and your program is more flexible!
-
If you want to re-use that login screen on a new piece of functionality,
you simply write a new object. You don't have to rewrite the login
screen.
-
Say you are writing a travel web site. If the user enters the site
and hits the 'Login' button, an object is passed with a login() implementation
that renders the user's home page. On the other hand, if the user
does not log in, but goes to purchase a ticket, the site might prompt the
user to login before making a purchase. This screen would pass an
object with a login() implementation that opens the checkout screen.
-
The patterndepot text gets into inner classes an anonymous inner classes.
This gets confusing. Let's forget about that and look at an example
we've already seen.
-
As a matter of fact, this example uses elements of command, singleton,
and factory patterns.
-
In the jukebox example, the tricky part about
using the if test is creating a new object of the song type that the user
selected. Many people left this out, it is a common error.
-
Basically, the jukebox had a global variable of type Song. Many people
would assign the song to a specific Song subclass when a JRadioButton corresponding
to that subclass was chosen.
-
For example, if the user chose the radio button for Disco, a new Disco
object was assigned to the global variable of type Song.
-
Then, when the user clicked 'Add', this object was populated with Artist,
Title, and Type.
-
What if the user added another Disco song immediately after the first Disco
song? Then a new object was never created, the same object was re-used!
This would overwrite the previous song.
-
So, the Song subclasses had to be smart enough to create instances of themselves.
After the song was added, the Song global variable had to be assigned to
a new object, like so:
song = song.getNew();
-
Now, getNew() had to be declared as an abstract method in class
Song, so that we could call it from Song. We further had to define
that in the subclasses, so that calling getNew() would actually return
an instance of that subclass. For instance, in Disco, we have:
public Song getNew() {
return new Disco();
}
-
While in Classic, we have:
public Song getNew() {
return new Classic();
}
-
Alas, the theory of the command pattern:
-
The calling class does not know the details of the
behavior it is calling. That behavior is handled where it belongs,
in the class that performs the decision making. In other words, deciding
to instantiate a Disco object is left up to the Disco class, not the Jukebox
class. The Jukebox class is simply for presentation, not decision
making!
-
And once again, we've managed to instantiate a new
subclass without resorting to a nasty if test.
Structural Patterns
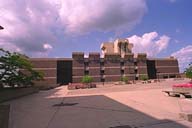
Created by: Brandan Jones
December 17, 2001