Class 2 |
Intermediate Java 30-IT-397 |
|
Rethinking the Jukebox Program
-
Last quarter, the final assignment was to make a Jukebox
interface.
-
There are many ways to do this. As you may recall, I gave extra credit
if you were able to write the entire program without using an if test.
One way to do that is with one listener for each type of music.
-
We can do the same thing with only one listener, and a factory.
-
Each JRadioButton has the same listener attached.
-
The listener gets the label from the JRadioButton, and passes it to a factory
method.
-
The factory method then returns an instance of that subclass.
-
We need to introduce a few new concepts to make this work.
-
First, we still need to know what song type the user clicked. We
can get this from the Event object that we pass to the listening method
- evt.getSource() will return an instance of the calling object.
-
Then, we need to cast that to a JRadioButton type, since a JRadioButton
initiated that event.
-
Now, we need to get the text label out of that JRadioButton so that we
know what the user clicked. We can use the getLabel() method of the
JRadioButton class.
-
Once we know the label of the button that the user clicked, we can pass
that to our factory method, songFactory(String songType).
-
Our factory method can instantiate that class dynamically. The Class
class has a method, getClass, that will get a class based on the String
passed to it. newInstance() will actually create a new object instance
of that class.
-
We cast that object to a Song type and return it.
-
Using getClass() and newInstance requires a try-catch block, which we'll
cover later today.
-
Take a look at the original source of all classes
used.
-
Take a look at the new source with the factory
method. Changed text in green. Only the Jukebox class changed.
-
What kind of class diagram would you draw for this?
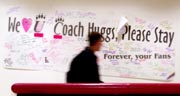
Created by: Brandan Jones
December 17, 2001