This site provides information for the classes I teach at Clermont College, The University of Cincinnati and courses I taught in another life.
Office: 3314 Lindner Hall
eMail: nicholdw at ucmail dot uc dot edu
Phone: (513) 556-7127 (email is preferable. I may not check office voicemail on a daily basis)
Office Hours: Spring 2025 schedule
Teaching Schedule: Spring 2025 Schedule.
MS Teams: 'call' me @ nicholdw during office hours
|
IT 1090/1091 Computer Programming I,II (formerly CIS 260/261/262 )
- Supplemental resources for this course
Computer Architecture Lecture and Lab
- Lecture / Lab Syllabus
- Supplemental resources for this course
OOP with C++
- Syllabus
- Supplemental reading for this course
Unix System Administration Lecture and Lab
Operating Systems Lecture and Lab
Useful Links
Reading List
Coding Standards for Higher Level Languages
Symbol Names
- Philosophy
- Symbol names should be consistent, reasonable, and meaningful
- "Consistent" implies that naming conventions you use at the beginning of a project should be carried through to the end of the project
- "Reasonable" implies that symbol names should not be too short, too long, too obscure, or offensive in any way
- "Meaningful" implies that the name should provide basic information about what the symbol represents
- Hungarian Notation
- You can use Hungarian Notation, or not. I encourage using it it because even if you don't adopt it for the rest of your career, you will see it in common use throughout the programming profession. Eventually you will be asked to maintain existing code that was written in this style.
- Wikipedia
- Dr. Charles Simonyi
- Funny Stuff
Modularity (FMSP = Functions / Methods / Subroutines / Procedures)
- Philosophy
- FMSPs should be short and easily understood by other programmers
- Several smaller FMSPs are better than one monolithic FMSP
- All FMSPs should...
- Exit out the bottom and only out the bottom, unless throwing an exception
- Use local variables whenever possible. Global variables are generally a bad thing.
- Be no longer than one 'page' (or one screen)
- Be independent of any forms and I/O devices whenever possible
OOP Classes
- Philosophy
- All classes should be written and maintained with an eye toward reuse.
- Consider your audience when writing a class
- Every class should be in a namespace. Multiple classes that are logically related and/or dependent can be placed in the same namespace.
- No global or public variables in classes: provide an appropriate Get/Set interface if necessary
- If a class does not need to be dependent on a form, don't make it dependent on a form.
Style Resources
Documentation
- Philosophy
- Software is written once and read many times
- In a small way documentation is for the original author, but it is mostly for those who will maintain the program down the road
- When you document software, put yourself in the shoes of the developers who will come after you:
- Assume they will have the same level of knowledge as you do
- What will they need to know about your work?
- What algorithms are unique to your work?
- What outside sources will they need in order to understand and maintain your code?
- What will not be obvious to them?
- If you came back to your code in 1 year, what things would you need to re-learn?
- Provide a comment header, or Flower Box, at the top of each module and disk file
- Name
- Course Number and Name
- Assignment Number
- Due Date
- File Name
- Abstract (the purpose of the module distilled to one or two sentences)
- References (web sites, books, people who provided algorithms that are used in the code)
- Change History (There probably won't be anything here, but add a label for it anyway)
- Provide a comment header at the top of each FMSP
- Use the default .Net XML tags if Visual Studio supports it
- Add more tags as necessary
- Use descriptive variable names, preferably some form of Hungarian Notation
- Do not use the goto command (unless you are stuck in VB 6)
- If your code cries out for a goto, you need to rewrite the offending logic and implement an FSMP instead.
Design
- Philosophy
- Some design exercises will seem redundant or overly simplistic. They may well be. Sometimes it's just "design for the sake of design." You are developing good programming habits and you are proving that you can design something.
- Provide a design document for all algorithms
- Use Visio or Word to create a flow chart for each algorithm
- The general purpose of the flow chart is to provide a definition of an algorithm
- The flow chart should include:
- names and data types of all symbolic references that the algorithm will use
- all formulas
- all decisions
- all input
- all output
- Note: The flow chart is not specific to any programming language.
- Use generic data types (integer, string, float, array, etc)
- Do not reference FSMP names that are specific to a programming language unless they are absolutely necessary.
- Do not write code in the flow chart: no delimiters, no language-specific syntax, etc.
- Use a CASE tool to create a class-level design
Program Architecture
- Philosophy
- You are writing a class, or multiple classes, that will be used by other programmers in their projects.
- Your classes must be independent of your main( )
- Your classes must compile even if your main( ) is not present
- Your classes must operate properly when integrated into other programmers' projects.
- Each class that you create should have two files associated with it:
- Both files typically have the same name as the class. In this example the class name would be myClass
- myClass.cpp is the source code for the class
- myClass.h is the declaration of the structure of the class
- Each project that you create should have a main( ) function associated with it
- The main( ) function should be in a file called main.cpp.
- Use conditional compilation to allow the main( ) to be easily excluded from the project
- #define __TEST_MAIN__
- #ifdef __TEST_MAIN__
- // main( ) goes here...
- #endif
- The main( ) function is referred to as a test main
- Set up the test environment by initializing variables, creating test data, prompting the user, etc.
- Invoke the class appropriately
- Evaluate the results and create an appropriate display for the user. The display should be clear and complete. Don't make the user guess what happened. Don't obligate the user to perform any calculations or comparisons.
Stuff I've Worked On
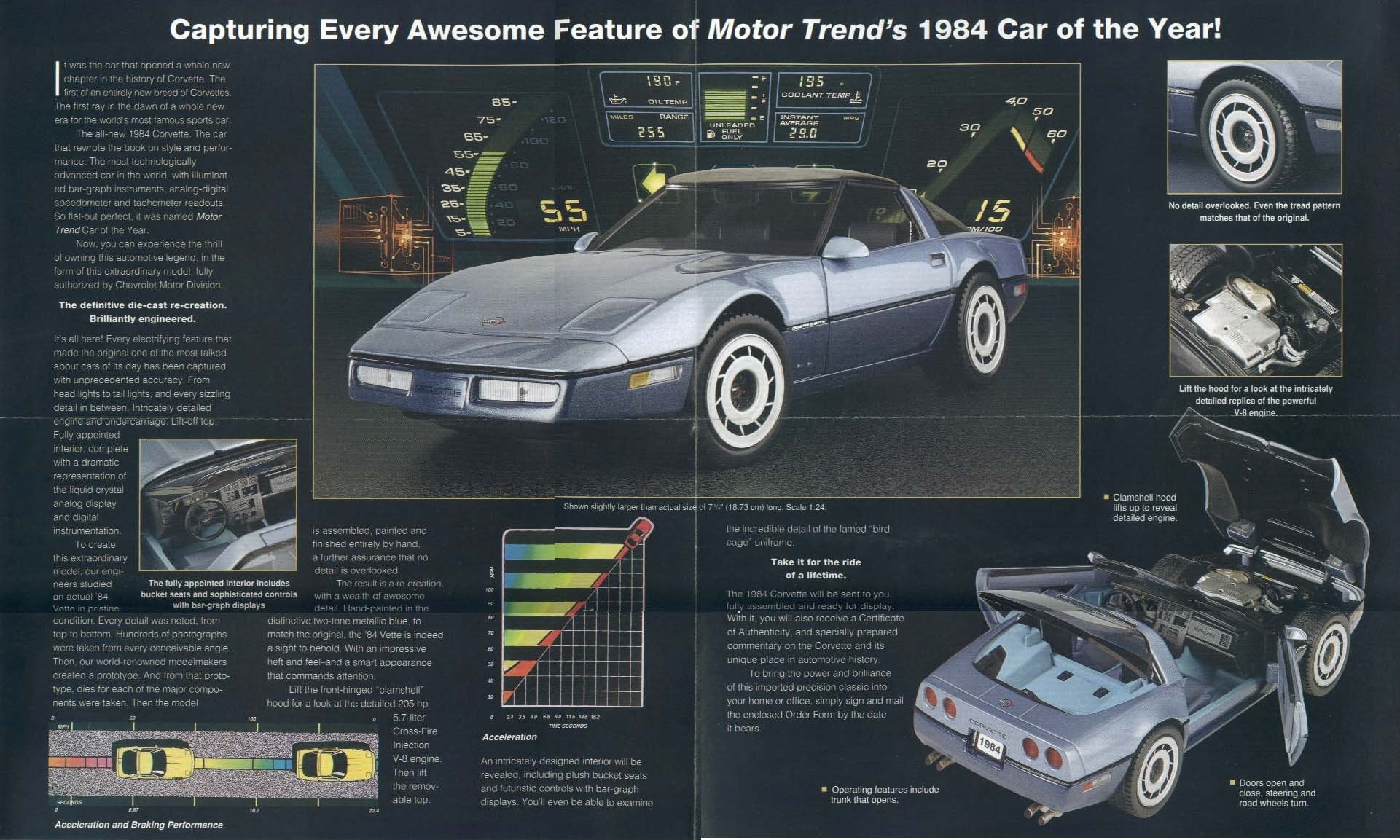
Note the high-tech digital dashboard.
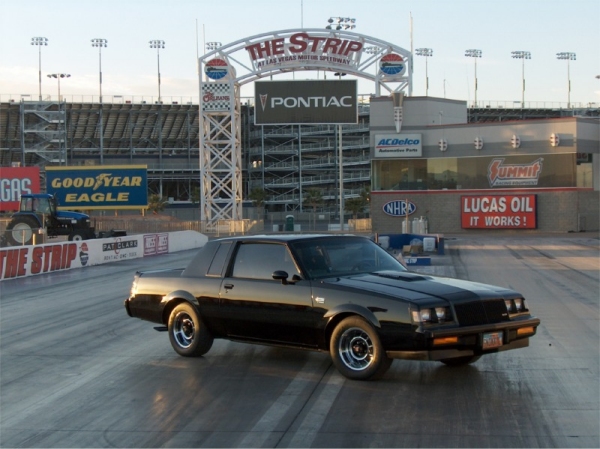
Note the Balloon Tires.
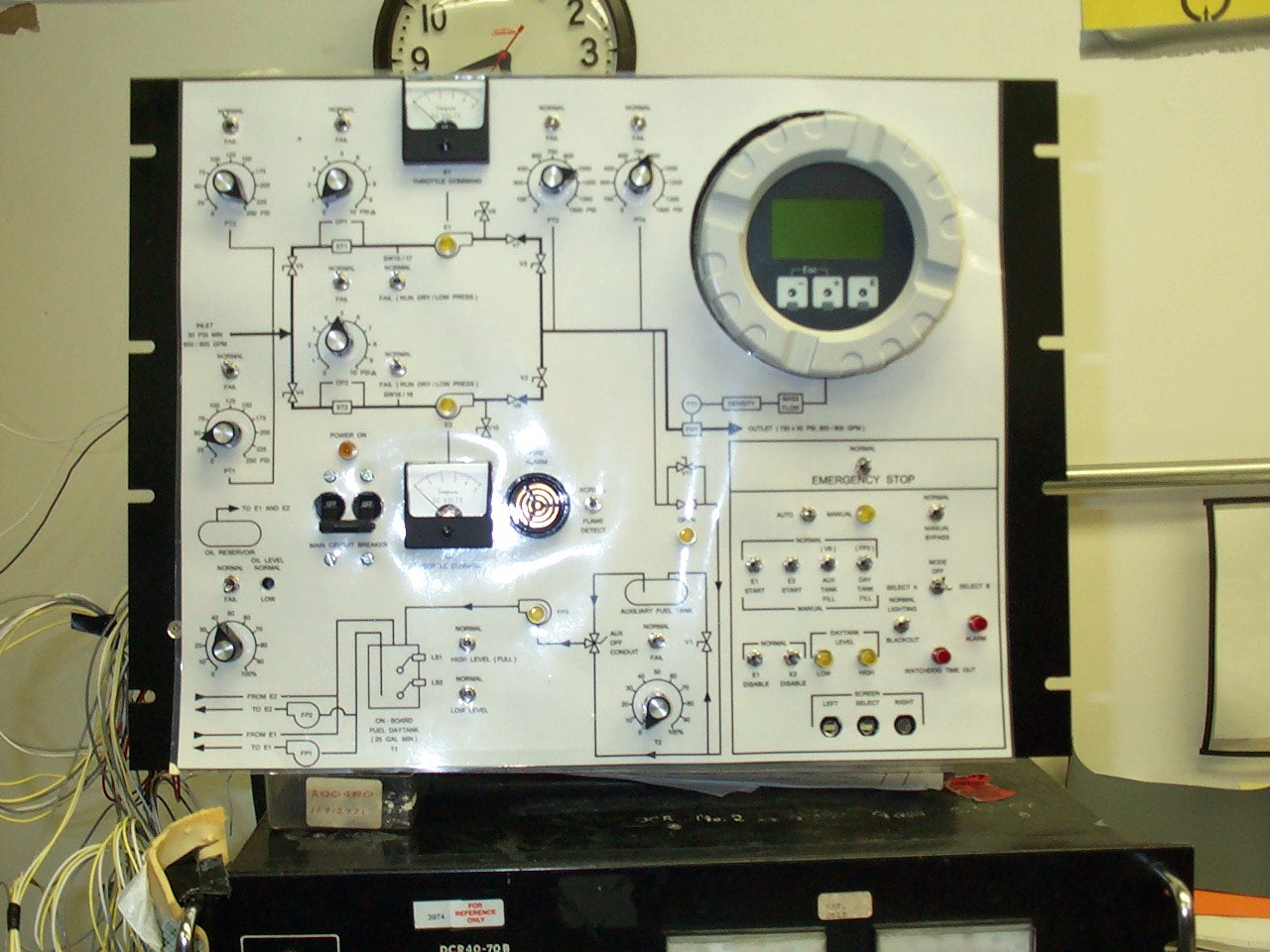
Simulator.
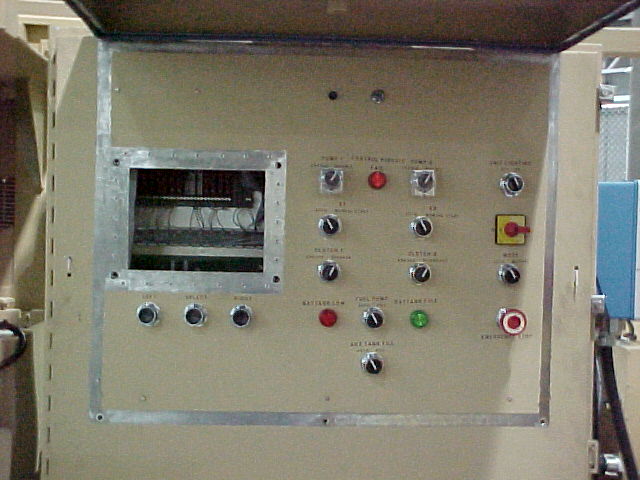
Front Panel.
- Blooms's Digital Taxonomy
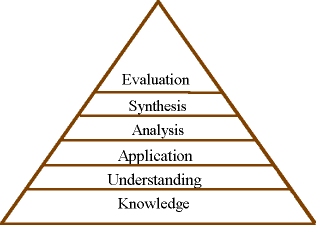
In 1956, Benjamin Bloom headed a group of educational psychologists who developed a classification of levels of intellectual behavior important in learning. Bloom found that over 95 % of the test questions students encounter require them to think only at the lowest possible level...the recall of information.
Bloom identified six levels within the cognitive domain, from the simple recall or recognition of facts, as the lowest level, through increasingly more complex and abstract mental levels, to the highest order which is classified as evaluation. Verb examples that represent intellectual activity on each level are listed here.
- Knowledge: arrange, define, duplicate, label, list, memorize, name, order, recognize, relate, recall, repeat, reproduce state.
- Comprehension: classify, describe, discuss, explain, express, identify, indicate, locate, recognize, report, restate, review, select, translate,
- Application: apply, choose, demonstrate, dramatize, employ, illustrate, interpret, operate, practice, schedule, sketch, solve, use, write.
- Analysis: analyze, appraise, calculate, categorize, compare, contrast, criticize, differentiate, discriminate, distinguish, examine, experiment, question, test.
- Synthesis: arrange, assemble, collect, compose, construct, create, design, develop, formulate, manage, organize, plan, prepare, propose, set up, write.
- Evaluation: appraise, argue, assess, attach, choose compare, defend estimate, judge, predict, rate, core, select, support, value, evaluate
- Five Dumb Arguments that Smart People Make
- News from the correlation-is-not-causation department
The Cherry Theorem (If you think you know the solution, please email it to me and I will credit you here.)
Q: What is a small, red, round thing that has a cherry pit inside?
Winners (?): Tony Hayes, Fall 2010
Chris Titzer, Summer 2014
Patrick Voto, Spring 2016
Connor McKinney, Fall 2019
Alexendria Gough, Spring 2020
Binta Drammeh, Fall 2023
Anthony Caggiano, Fall 2024
Selected Essays
Test Taking Tips
- First, read the whole thing.
Before answering any questions, read every question.
- Cherry Pick.
Answer the easy questions first. This will build your confidence and help you focus.
- Study the question before answering it.
Many questions have multiple parts. Be careful to answer all parts. For example:
"List the parts of a fuel injection system and explain why each part is important."
- Perform a sanity check on your answers.
Be sure your answer makes sense. Does it 'look' correct? Are your units proper? Do you have the correct number of decimal places? Does the answer match the question?
- Convince the grader that you know what you're talking about.
A one-word answer may be technically correct, but it may also be too vague.
- Don't edit the questions.
You didn't write the test. If you don't understand a question, ask for clarification. Don't be shy!
Free Software, although Richard Stallman may disagree.
People Who I am not
Enjoy Every Sandwich
|