Java: How Do I?
I frequently get numerous student questions starting with the words "How do I do _____?" The following list of
items is a compilation of those general questions along with their answers, arranged in no particular order.
Use your browser's search facilities to find the answers to your most pressing Java questions. Enjoy!
- resolve javac not found error - add javac directory location to Path variable. Open Windows search
for files or folders, enter javac. When the search tool returns the location of javac.exe, add this to the end of
the Path variable as: follows.
Note, prefix new directory addition with a semicolon.
- disable overwrite mode in NetBeans - turn num lock off, then use the 0 (insert) key to toggle between
insert and overwrite.
- display a missing window in NetBeans - click the Window dropdown toolbar of NetBeans, select IDE Tools, find the name
of the window you are missing, and click away. Note, palette and properties might be the most used/useful.
- resolve class Scanner not found - select Source toobar, Fix Imports, Fix All Imports, OK. Or, below
the package statement, type the following: import java.util.Scanner;
- resolve variable foo might not have been initialized compile error - manually set the variable to 0
or something zero like at declaration time, e.g. double foo = 0.0 ;
- print only the code I want, and not lots of empty pages - 1) in file, print, print options, check "wrap lines".
2) highlight only the code you want to print, then in file, print, print options, check "print selection".
- delete an empty, unwanted event - 1) delete the control object the empty event is tied to, and the event
should go away, then re-create the control object. 2) select the control object (here, jTextField1), click on the
events tab in the properties window, find the event you wish to remove, click the custom editor button
(with the 3 dots), and click Remove.
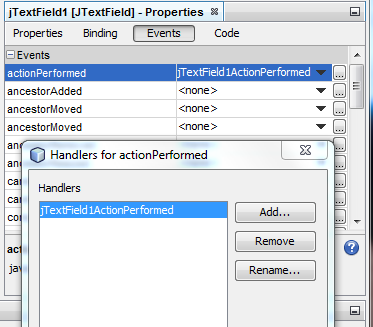
center main form - change the following code in your main method (inside the public void run () method):
new MainForm().setVisible(true);
to
MainForm frame = new MainForm();
frame.setVisible(true);
frame.setLocationRelativeTo(null);
set a button as the default - (so Enter triggers the event), in the constructor for the form, include the
statement
this.getRootPane().setDefaultButton(button_name);
Note: you can only have 1 of these set.
execute one event from another - use the .doClick() method, e.g. btnReset.doClick();
include a % sign in a printf statement - if you wish to include a simple % sign in a printf statement,
you must "escape it" using another % sign, otherwise a
java.util.FormatFlagsConversionMismatchException: Mismatched Convertor =s, Flags=
error will occur. For example:
System.out.printf ("This line prints a single %% sign.%n");
return more than one value from a method - simple answer...you can't. However, you can work around
this by returning an array of values, or returning an object which contains multiple values.
public static int[] blah(){
int foo = 1;
int bar = 2;
return new int[] {foo, bar};
}
handle divide by zero - dividing an int by 0 will result in a throwable exception, which can be
handled using an exception handler (catching an ArithmeticException). With floating point types, an exception
will not be thrown. The surest way is to check any denominators for values of zero before performing the
division operation. Otherwise, to check a resulting value following division, one can do the following:
double result = // division operation
if (result == Double.PositiveInfinity) { // likely need to check .NegativeInfinity also
See specification here.
©2018, Mark A. Thomas. All Rights Reserved.