Class 1, Part 2 |
Intro to Java 30-IT-296 |
|
30-IT-296 Assignment 1
Summer Quarter 2003 Note:
Updated Weds, July 9, 2003. Changed due dates
to reflect changes made in class on 7/10/03.
This is the assignment is divided into three parts, due at three times
throughout the quarter. These parts include:
-
Part 1 - Due Wednesday, February 4, 6:30 PM:
Described
below under the red heading Part 1.
-
Part 2 - Due Week 6: The assignment listed under
Part 2.
-
Part 3 - Due Week 8: (Not yet posted) The assignment
below as an applet. Also add exception handling.
I will post updates to this page in blue text.
Part 1, Due 7/24/03 6:30 PM
Write the structure for two classes (.java files).
The first class...
-
Name the first one LoanCalc.
-
Add three methods: promptUser, calculate, and main.
-
main should call the other two methods, and should
have the proper modifiers (example: public, private, static, final, int,
float, void, etc.) for a method named main, as discussed in class.
-
Add five variables (instance fields), named principal,
rate, numPeriods, remainingPrincipal, periodNumber. Choose the most appropriate
type.
The second class...
-
Name the second class Converter.
-
It should have two methods, one named calculate,
and the other named main. main should follow the same rules above, as discussed
in class.
-
It should also have two variables, one named celcius
and the other named fahrenheit.
Grading Criteria:
-
The classes compile (they do not need to work, just
compile).
-
In on time, by due date.
-
All methods are present.
-
All variables (instance fields) are present.
-
Variables have appropriate type.
-
Methods have appropriate modifiers.
-
Proper use of curly braces and parenthesis.
-
Source code in good form.
Notes:
Please upload your source files (.java files)
via
blackboard by Wednesday, July 24, 2003 by 6:30 PM. Late penalties
begin at 6:31 PM on Wednesday, July 24.
These classes do not need to do anything at this
point. Eventually, we will enhance them as detailed in part 2 below. But
for now, all I am looking for is the general structure of the classes -
proper use of methods, variables, curly braces, etc., as described above.
Feel free to add functionality in addition to the above specifications;
however, this is not required.
Part 2, Due Week 7
-
Lab time, either on campus or remote, will be offered if necessary.
Please IM UCInternet2 or email Brandan.Jones@uc.edu if you would like to
request assistance.
Part A:
Pretend you work for a bank. Your boss has asked you to make an online
savings calculator, where
customers can calculate the value of their money after interest compounds
for a number of periods. You must prompt the user
for a beginning principal (deposit amount), rate of interest per period,
and number of periods. You must then display, via System.out.println();
the balance at the end of each period. The interest must compound
once
at the end of the period (only once per period, don't compound more
than that).
Hints:
-
Use JOptionPane.showInputDialog("your prompt message here"); to gather
the three inputs, one prompt per input, for a total of three prompts.
Store the value of these prompts in variables.
-
Use a loop to iterate through each period. Inside that loop, use
this formula to calculate the ending balance:
-
principal = (principal * rate) + principal;
-
Don't forget, since interest compounds once per period, you must make the
principal at the end of the period equal to the principal at the beginning
of the period plus interest earned that period. In other words, use
the formula above, don't re-use the beginning principal amount each time.
The ending principal should increase over time.
-
You may need to convert variable types. For example, the inputs come
as Strings, but you will want to change them to floats or ints. Look
up the Integer and Float classes on the API
Documentation for more information.
After the above calculation, use System.out.println(); to print the balance
at the end of the period. Print one line per period.
For each period, print the period number, interest earned, and ending
balance.
Part B:
Use the command line arguments (or Properties - Execution - Arguments
in Sun ONE) to get a fahrenheit temperature from the user. If no
temperature is provided from the command line, use a default of 35.
Compute the celcius equivalent, using the formula below, and print it to
standard output via System.out.println();
Celsius
= (Fahrenheit – 32)*5/9
Hints (Updated for clarity, 8/11/2003):
-
Do not use JOptionPane.showInputDialog to get the temperature. Instead,
set the temperature with the command line or the Sun ONE properties window,
described above. Then, get the temperature as the first element in the
args array.
-
You'll need to test the array length to see if a temperature has been passed
in via args. If so, use hint #1 above to get the temperature. If not, use
the default value 35 fahrenheit to calculate the celcius equivalent temperature.
-
If you attempt to access an array element that does not exist, you will
get a NullPointerException. If no arguments are passed and you attempt
to get the first element of the array, you will get this error. The test
described in hint #2 above should make sure this never happens.
-
See the HelloWhirled example
from the first class for a refresher on using args.
Grading Criteria:
Overall:
Did something extra, something beyond the minumum requirements stated
here: 10
Commented: 10
Assignment 1:
Calculations are correct: 10
Prompts are correct: 10
Proper use of loop: 10
Proper creation and formatting of table: 10
Assignment 2:
Calculations are correct: 10
public static void main(String args[]) method is set up correctly,
and fahrenheit temperature is properly retrieved from command line: 10
Default temperature is used if none are set in the command line, otherwise
the command argument is used: 10
Proper conversion of data types: 10
Additional Deductions:
Code not in good form (sloppy, bad spelling, etc.): up to -10
Late: -10% at the beginning each 24 hour period, starting promptly
at 6:30 PM on the due date.
Copying someone else's work: F for the entire quarter.
NO credit will be given for assignments more than four days late.
Part 3, Due Week 9
Update 8/15/2003: Clarified assignment with more
strikethrough. New text in blue.
Update 12/3/2003: Eliminated
strikethrough.
Lab time, either on campus or remote, will be offered if necessary.
Please IM or email ucinternet2@yahoo.com if you would like to request assistance.
Part A:
Using the existing LoanCalculator, make a class hierarchy that
contains the following classes:
Class Account
attributes (instance fields, variables) should have at least:
-
principal
-
rate
-
period
-
period number
methods should include at least:
Class Savings extends Account
Should have these attributes (instance fields, variables) or have
access
to them via class Account:
-
principal
-
rate
-
period
-
period number
methods should include at least:
Class Checking extends Account
Should have these attributes (instance fields, variables) orhave access
to them via class Account:
-
principal
-
rate
-
period
-
period number
-
check number
-
monthly fee
methods should include at least:
Class CertificateOfDeposit extends Account
Should have these attributes (instance fields, variables) or have
access
to them via class Account:
-
principal
-
rate
-
period
-
period number
-
length (to maturity; in months)
methods should include at least:
Class Banker
-
Prompt the user for an account to open - Checking, Savings, or CD.
-
Prompt for beginning balance, interest rate, length. Pass these to
the constructor of the appropriate object which you are creating.
So, each object should have at least one constructor that takes these three
variables: beginning balance (principal), interest rate (rate), length.
-
If checking, prompt for first check number and store. Make fee =
5.
-
If CD, prompt for length until maturity.
-
Repeat the process - ask if the user would like to open another
account, until the user types stop (or something else that indicates that
he/she is finished).
-
Save each account in a List of some type an array, ArrayList, or
Vector.
-
When finished, call the calculate method on each account. Display the same data described
in Part 2 above.
-
If the account is a checking account, subtract the monthly fee from principal
on each period in which interest is computed.
Other Criteria
-
Add exception handing (try-catch) if the
user attempts to enter alphabetic characters in a numeric field.
(Changed 3/5/03: This is no longer a requirement. However, if you would
like to do this, it will count as an 'extra' feature.)
-
Validate data the user enters.
-
Interest Rate may be no greater than 25% (as a decimal or whole number,
depending upon how you collect data).
-
Check number cannot be negative.
-
Do something extra.
-
Comment
Grading Criteria:
Overall:
Did something extra, something beyond the minumum requirements stated
here: 5
Commented: 10
Prompt user to create an account, and allow the user to choose from
the three (or more): 10
Proper use of inheritance (extends, subclassing, etc.): 5
Checking has check number and monthly fee fields, has proper implementation
of calculate() and CertificateOfDeposit has lengthToMaturity. These
fields are not available in other classes: 5 10
Store accounts in a list of your choosing (array, ArrayList,
Vector, etc): 10
Calculations are correct: 10
Proper use of loop: 10
Proper data output: 10
Exception Handling (try-catch): 10 (Changed to optional 3/5/03 bj)
Data Validation: 10
Constructors set up properly: 5
Additional Deductions:
Code not in good form (sloppy, bad spelling, etc.): up to -10
Late: -10% at the beginning each 24 hour period, starting promptly
at 6:30 PM on the due date.
Copying someone else's work: F for the entire quarter.
NO credit will be given for assignments more than four days late.
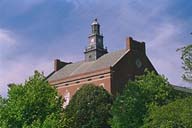
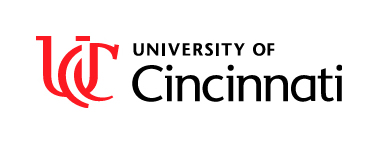
Created by: Brandan
Jones January 23, 2002