Class 2 |
Intermediate Java 30-IT-397 |
|
Cookies, the old fashioned way
-
For old time's sake, let's make a JSP with cookies.
-
We'll need the Cookie class and response object. The Cookie class
is included in the packages we already import, so no extra work is needed
there. The response object is included in JSPs, so we still don't
need any extra effort.
-
We also have these resources available to us in a servlet, so we can use
this same example there.
-
Normally, a cookie would have a unique ID that would relate back to a database
entry or complex customer account information. But, we're not that
complex at this point. So let's just store the user's name.
-
How we do it:
-
We need to make an instance of the Cookie class. The Cookie class
accepts a name and value as parameters ot its constructors.
-
We can use request.getCookies() to get an array of cookies stored, and
response.addCookie (why not setCookie?) to set the cookie on the user's
computer.
-
Putting this all together...
-
We can have an HTML form that accepts the users name and calls itself when
submitted.
-
When called the second time, it takes this parameter out of the request.
If the parameter is populated, it sets this information in the cookie.
Note that it checks to see if the parameter is null. If so, it will
not set the cookie.
-
The next time around, it gets the cookies, and looks for the one with the
user's name. When it finds that, it prints out a welcome message.
-
The source:
<%@page contentType="text/html"%>
<html>
<head><title>JSP Page</title></head>
<body>
<%-- <jsp:useBean id="beanInstanceName" scope="session" class="package.class"
/> --%>
<%-- <jsp:getProperty name="beanInstanceName" property="propertyName"
/> --%>
<%
// Get an array of cookies that exist.
Cookie cookies[] = request.getCookies();
// Loop through that array.
for (int i = 0; i < cookies.length; i++) {
// If we find one with a name userName, print
a welcome greeting.
if (cookies[i].getName().equals("userName"))
{
out.print("Welcome "
+ cookies[i].getValue());
}
}
// Get the name, if passed in.
String name = request.getParameter("name");
// Write the name to a cookie for the next refresh.
if (name != null) {
Cookie cookie = new Cookie("userName", name);
response.addCookie(cookie);
}
%>
<form name = "welcome" action = "cookiesjsp.jsp" method=GET>
What's your name, dude?
<input type = "text" name = "name">
<input type = "submit">
</form>
</body>
</html>
Newfangled Sessions
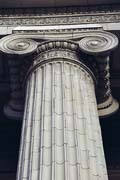
Created by: Brandan Jones
December 17, 2001