Class 2 |
Intermediate Java 30-IT-397 |
|
Thread States
-
Threads have a lifecycle, as do many programs and Java objects. At
any point in time, threads can be in one of four states:
-
new
-
runnable
-
blocked
-
dead
-
New
-
When you instantiate a Thread object, it is in the new state. It
is not yet running, it is simply an object that can be run when
called.
-
Runnable
-
A thread is runnable when the start() method has been called. It
might not be running, but at this point, it can run. When the operating
system gives the code time to run, it is then running.
But, when it is running, it can yeild control or be stopped by the operating
system so that another thread can run. At this point, it is no longer
running.
-
Some operating systems use time slicing with threads, where each thread
is given a slice of time to run, so that no single thread can hog the entire
CPU.
-
Blocked
-
A thread is blocked after it starts running, but when it is not actually
running. What causes a thread to block?
-
Calling the sleep() method of the thread.
-
Waiting for input or output, from the user, a URL connection, disk, etc.
-
Calling the wait() method of the thread.
-
Waiting for a lock on another object.
-
After the thread is blocked, it will run if it is the thread with the highest
priority in the Runnable state.
-
Threads come out of the blocked state when:
-
The sleep interval has expired.
-
The I/O operation has completed.
-
notify() or notifyAll() is called when the thread is in the wait() state.
-
If the lock on an object has become available.
-
A thread can only leave a blocked state for the corresponding reason that
blocked it.
-
Dead
-
When a thread dies, it cannot run again.
-
Threads die because the run() method is simply finished and terminates,
or an exception is thrown.
-
You can always kill a thread when interrupt() is called by throwing an
exception that terminates run()..
-
isAlive() will return true if a thread is still alive.
Daemon Threads
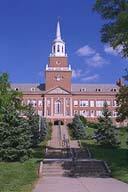
Created by: Brandan Jones
December 17, 2001