Class 2 |
Intermediate Java 30-IT-397 |
|
Example
-
Let's make a simple Swing application to demonstrate Strings with JLists.
-
This is the most straightforward approach, and probably the most familiar
to you. But is also frowned upon in the world of OO.
-
We'll have a JFrame object that has:
-
A JList that contains Strings that represent building names.
-
A JScrollPane to allow the JList to scroll.
-
A JButton for the user to submit values selected on the JList.
-
A JOptionPane that pops up when the user clicks the JButton.
-
A Building array that contains all of the possible Building objects (see
below).
-
In addition, we'll have a Building class that represents buildings on campus.
-
This class will have two attributes - buildingName and primaryUse.
-
The user will select buildings from the JList in which he or she has visited.
Then, the user will click on the JButton and the JOptionPane will pop up
that describe possible activities that the user performed in those buildings.
-
In Forte'....
-
Create a new JFrame object by choosing New-GUI Form-JFrame. Give
your JFrame object a name, and click Finish.
-
Open the JFrame in the GUI Editor. Add a JScrollPane to the CENTER
part of the JFrame's layout. Then, add a JList to that JScrollPane.
Add a JButton to the SOUTH section.
-
You can nest these within panels if you want more control over the layout.
-
All of these components can be found on the menu with the Swing tab.
-
Add a title, if you wish.
-
Change the names of the objects to something more meaningful.
-
Now, add some logic.
-
In the source code, go to the constructor.
-
Make an array of building objects.
-
Make a similar array of names of the building objects, via getName().
-
Add this String array to the JList. Note that, since Forte' automatically
creates the JList for us and locks it down, we have to use the setListData(Object
array[]) method to set the data, we can't use the constructor.
-
Back to the GUI Editor...
-
Right-click on the JButton, and add an ActionListener.
-
When Forte' brings you to the source code, use the getSelectedItems() method
of the JList object to get the selected items.
-
Iterate through the object array returned, cast each element to a String,
and then iterate through the building array to find the building that was
selected to the list.
-
Add each selected building function to a String object that you pass to
JOptionPane.showMessageDialog.
Source Code and Screen Capture
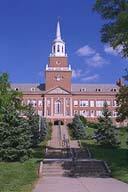
Created by: Brandan Jones
December 17, 2001