Class 1, Part 2 |
Intro to Java 30-IT-396 |
|
Swing and the Model-View-Controller
Pattern
-
Swing is Java's way of making GUIs, since version 1.2.
-
Before that, Java used the Abstract Windowing Toolkit, or AWT.
-
AWT served its purpose, but is not a good object-based interface.
-
Swing follows the rules of object oriented programming by separating the
view of the component from the model, or data, of it. Think of the
view of a button or a checkbox, versus the data that it represents.
In Swing, these are different objects.
-
More specifically, the Model View Controller design pattern says this:
-
The model is the data that the component (text field, radio button, drop
down, etc) represents.
-
The view is how the component looks on the monitor.
-
The controller handles the behavior of the component, or how it interacts
with the data that it represents.
-
Sound confusing? I am not surprised. But it's not so bad when
you think about it.
-
Remember our car with the go() method? Say this method is activated
by a button. It wouldn't be a good idea to have the go logic in the
button, would it?
-
Furthermore, suppose there is more than one way to activate that go().
We would want to reuse that logic among the different ways to activate
go(). We wouldn't want to write all of the logic for each different
way.
-
Think of another example. Suppose we wanted to have a drop down list
with all of the car models that we can choose. Wouldn't it make more
sense to pass in an object, as a model, that has those car models instead
of send each one as text? The class we pass in might as well be the
same class that the Car class uses when it references model information.
-
And it is actually not as bad as it looks. The model and the view
are usually integrated together in Java, so you don't have to worry about
them. All you need to be concerned with is getting the data to the
component, or from the component.
-
Most swing objects, such as JButton and JTable, store a model object.
If necessary, you can get this model from the swing object, but you usually
don't have to.
Streamers
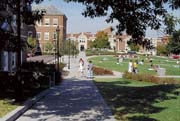
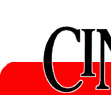
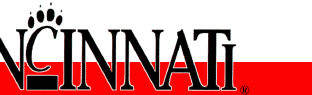
Created by: Brandan
Jones January 4, 2002