Class 1, Part 2 |
Intro to Java 30-IT-396 |
|
More About Arrays
-
If you know the values of an array ahead of time, you can assign them
to
the array when you declare the array.
-
Just put the values in curly braces after the =. Separate the
values
with commas. Example:
String[] sanderLabs = {"L203", "L206", "L209", "L221", "L222"};
-
You can even assign this new array to an old array, and the size of the
array will dynamically change to reflect the size of the new array.
-
Example:
names = sanderLabs;
- At this point, names no longer has the size 34, but 5
instead.
-
Just make sure the types are the same.
-
A more sophisticated copy:
-
You can copy elements from a specified position in one array to a
specified
position in another.
-
Since arrays are not objects, per se, you have to use a System
method.
-
System.arrayCopy(from, fromIndex, to, toIndex, count);
-
Where:
-
from is the from array.
-
fromIndex is the first element to copy.
-
to is the to array.
-
toIndex is the position to which to copy the elements.
-
count is the number of elements to copy.
-
Example: To copy the middle three rooms from sanderLabs to names,
starting
with the fifth element in names:
System.arraycopy(sanderLabs, 1, names, 4, 3);
Even More About Arrays
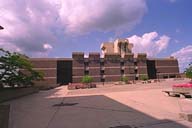
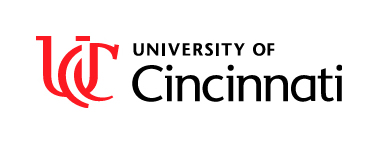
Created by: Brandan
Jones January 4, 2002