Class 2 |
Intermediate Java 30-IT-397 |
|
In Class Exercise
-
This exercise uses UML, Design Patterns (Command), and Exceptions.
-
You need a few classes.
-
Class WhatDoYouWant is your controller.
-
It has public static void main(String args[])
-
It takes an input parameter. This parameter can be Hamburger, Fries,
Fresca, or Tab.
-
It calls a method, takeOrder(String args[]). takeOrder instantiates
objects that inherit from class Item. You can either use an if test
to determine what to instantiate, or use
Class.forName().newInstance(), whichever you prefer.
-
Class Item is an abstract superclass.
-
It has one abstract method, askQuestion();
-
Class Hamburger extends Item.
-
Its askQuestion implementation has:
-
System.out.println("rare, medium, meduim well, or well?");
-
Class Fries extends Item.
-
Its askQuestion implementation has:
-
System.out.println("Light or heavy salt?");
-
Class Fresca extends Item.
-
Its askQuestion implementation has:
-
System.out.println("Regular or Diet?");
-
Class Tab extends Item. It implements askQuestion, but the implementation
is empty.
-
When the user runs WhatDoYouWant with one of the above parameters, WhatDoYouWant
should send the args[] array to method takeOrder(String args[])
-
This method must instantiate the object represented in the args[] array.
You only need to instantiate one, but you can handle multiple objects if
you wish.
-
If the argument is Tab, you must throw an exception. This code is:
-
throw new Exception ("Are you serious?");
-
public static void main(String args[]) must have a catch block to handle
this exception and print it out.
-
For all other objects, run the askQuestion() method. Hence, the Command
Design Pattern!
-
Before you write any code, make the UML. Either with Poseidon or
on a piece of paper.
-
This is a simple example. Use if tests if you like, I won't mind.
If you finish early, perhaps add extra functionality (ability to handle
multiple arguments, for instance.)
-
Ask me if you have questions.
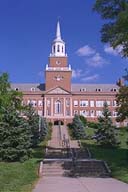
Created by: Brandan Jones
December 17, 2001