Class 2 |
Intermediate Java 30-IT-397 |
|
JavaBeans and JSPs
-
We've talked a lot about JavaBeans. Now they are finally going to
make our lives easier.
-
First a review:
-
JavaBeans are simply Java classes that have private instance fields, or
attributes.
-
These fields are accessed via public getter and setter methods. These
methods control access to the variables.
-
JavaBeans have a zero-argument constructor.
-
JavaBeans should be used whenever possible. It's really a good coding
methodology.
-
Now, using them with our JSPs.
-
Use the <jsp:usebean id="name" class="package.class" scope="scope"
/> tag to use the bean in the JSP.
-
This instantiates the bean named in the class argument to a variable with
the name in the id argument.
-
Scope is optional; the values permitted are outlined on page 303 of the
book/
-
This tag uses the XML syntax, so the attributes are case-sensitive; quotes,
whether single or double, must be used; and the tag ends with />
-
Once we've added the first tag, we can add the following tags to get or
set values in our beans:
-
<jsp:setProperty name="name" property = "property" value = "value"
/> sets the value of the value parameter to the property (setter method)
specified in property for the bean named in name. In other words,
name.setProperty(value) in this example.
-
Alternatively, <jsp:setProperty name="name" property = "*" />
automatically sets the properties in the bean where the bean properties
match the incoming request parameter properties. Wow! You only
need one of these to call all of those getters.
-
<jsp:getProperty name="name" property="property"> calls the
getter method matching the property value in the bean named in name.
In other words, name.getProperty().
-
As you can see, this is makes our application much more object oriented.
Let's re-engineer our previous application to take advantage of this.
-
Student.java and sessionshtml.html do not change.
-
For the others, we completely eliminate our references to session,
because our bean will be a session bean.
-
We simply add the useBean, setProperty, and getProperty tags.
-
sessionsexample new source
<%@page contentType="text/html"%>
<html>
<head><title>JSP Page</title></head>
<body>
<%-- <jsp:useBean id="beanInstanceName" scope="session" class="package.class"
/> --%>
<%-- <jsp:getProperty name="beanInstanceName" property="propertyName"
/> --%>
<jsp:useBean id="student" class="jsp.Student" scope="session"
/>
<jsp:setProperty name="student" property="*"/>
Congratulations <%= student.getName() %>, your registration is
complete!
<br>
<a href = "beanresults.jsp">Continue</a>
</body>
</html>
<%@page contentType="text/html"%>
<html>
<head><title>JSP Page</title></head>
<body>
<%-- <jsp:useBean id="beanInstanceName" scope="session" class="package.class"
/> --%>
<%-- <jsp:getProperty name="beanInstanceName" property="propertyName"
/> --%>
<jsp:useBean id="student" class="jsp.Student" scope="session"
/>
Your data:<BR>
Name: <jsp:getProperty name="student" property
= "name" /> <BR>
Year: <jsp:getProperty name="student" property
= "year" /> <BR>
Degree: <jsp:getProperty name="student" property
= "degree" /> <BR>
</body>
</html>
-
Incredibly simple, isn't it?
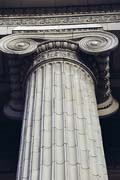
Created by: Brandan Jones
December 17, 2001