Class 1, Part 2 |
Intro to Java 30-IT-396 |
|
JComboBox
-
A JComboBox allows the user to select a value from a drop-down box.
-
This is similar to the JCheckBox and JRadioButton in that we provide the
user with predefined values.
-
But, JComboBoxes have an advantage because they can display a lot of choices
in not a lot of room. When the user clicks on the JComboBox object,
a drop down list appears, sometimes with a scroll bar, with all of the
options.
-
It is easy to dynamically populate a JComboBox with values. With
JRadioButtons and JCheckboxes, you often have to know all of the values
ahead of time and hard code them into the form. Not so with JComboBoxes,
these are often fed from a database. Just call the addItem(Object
item) method to add new items dynamically.
-
And, you can make JComboBoxes editable. That is, you can allow users
to type in their own values if the value they want is not on the list.
-
Suppose, for example, you had a JComboBox for a City field in the form.
Here it would be easy to add common cities, like Cincinnati, Norwood, Covington,
Newport, Blue Ash, Fairfield, etc. But what about a user who lives
in a township? Or a user who lives in Lawrenceburg? If that
option is not on the list, the user can simply type it in. Use the
setEditable(boolean b) method to do just that.
-
Use the getSelectedItem() method to get the currently selected
item.
-
An object-oriented note:
-
The book does it the quick and dirty way. It shows adding String
objects and retrieving String objects from the JComboBox. But if
you look at the Javadoc
for JComboBox, you will see that the methods actually deal with objects.
Since all objects extend class Object, which has the toString()
method, the JComboBox simply runs toString() on these objects.
But it doesn't stop there. You can also send an array or vector of
objects in the constructor, and you can send any object you wish in the
addItem(Object item) method. When you call getSelectedItem(),
you will get exactly that object back, not a String.
-
So what's the big deal? That means that you don't have to set up
a grand if test, based on a String returned from getSelectedItems(),
to find the object that was selected. You can simply add that object
in its raw form, then get the object itself back! This saves a lot
of coding and if tests.
Borders
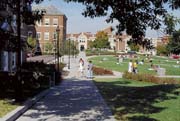
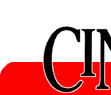
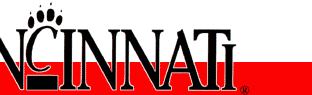
Created by: Brandan
Jones January 4, 2002