Class 1, Part 2 |
Intro to Java 30-IT-396 |
|
More about Polymorphism and Dynamic
Binding
-
To use Polymorphism, the classes must have an "is-a" relationship.
-
A Hybrid is a Car, a Car is a Vehicle.
-
In Java, an object variable of type Vehicle can refer to an object of type
Vehicle, Car, Hybrid, or Cavalier. In other words, it can refer to
an object of its own type, or one of its subclasses.
-
However, you cannot set an object variable to be the value of the superclass
of that variable type, at least without casting it. Why is that?
A subclass is guaranteed to have all of the instance fields and
methods of its superclass. But a superclass does not have
all of the instance fields and methods of its subclasses. The superclass
is more general. Hence, the nature of polymorphism.
-
Example:
public void run(Vehicle v) {
// You cannot do this. What if the Vehicle
v is not a Hybrid???
// Class Hybrid has methods and attributes that
are specific to that class.
Hybrid hybrid = v;
}
-
As for dynamic binding, let's look at it from the perspective of the compiler.
-
The compiler looks at the type of object and the name of the method.
It gets a list of methods matching that name from the object. Keep
in mind, methods can have identical names as long as they have different
signatures.
-
Then, the compiler looks at the parameter types of the method call and
the available methods, and finds the best match (based on the signature
of the method). If no match can be found, an error is generated.
-
If the method is private, static, final, or a constructor, the compiler
can use static binding to tell exactly which method to call. If not,
dynamic binding must be used at runtime to determine which method to call.
-
In dynamic binding, the JVM must determine the method to call based on
the lowest subclass of the object. So, if we pass an object Hybrid
to a method that accepts objects of type Vehicle, the JVM will look for
the method stating with Hybrid, then Car, then Vehicle. As soon as
it finds a match, it executes. So, if we call go() on a Vehicle object
that is actually of class Hybrid, it will run the go() method in Hybrid.
On the other hand, if we pass it a Vehicle object of type Cavalier, it
will not find a go() method until it gets to class Vehicle, and then it
will run that method.
-
Here's where it gets good.
-
Java has a way of finding classes dynamically. This is an advanced
topic, let's save the details for later. But let's just say that
we have class Hybrid and class Cavalier in the same package. If we
were to add a class, call it Civic, to that package, and the Controller
class were smart enough to find this new class, we could use it without
chagning anything else.
-
This is the concept of forward-thinking code, and is made possible
by polymorphism and reflection. But again, we'll save that discussion
for later.
Final Classes and Methods
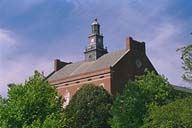
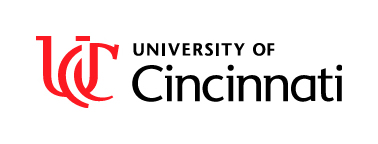
Created by: Brandan
Jones January 4, 2002